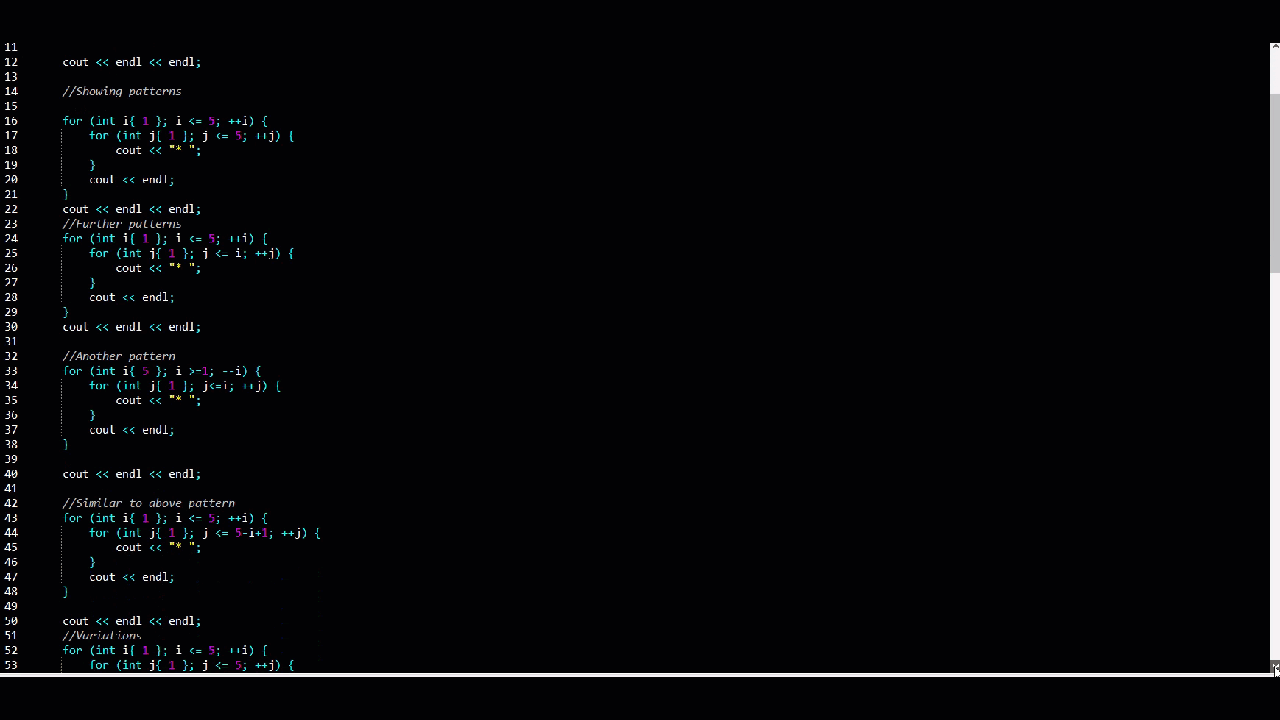
The following code is part of the pattern printing series:
This one will print a series of patterns
#include <iostream>
using namespace std;
int main()
{
for (int i{ 1 }; i <= 5; ++i) {
for (int j{ 1 }; j <= 5; ++j) {
cout <<"("<< i << "," << j<<") ";
}
cout<<endl;
}
cout << endl << endl;
//Showing patterns
for (int i{ 1 }; i <= 5; ++i) {
for (int j{ 1 }; j <= 5; ++j) {
cout << "* ";
}
cout << endl;
}
cout << endl << endl;
//Further patterns
for (int i{ 1 }; i <= 5; ++i) {
for (int j{ 1 }; j <= i; ++j) {
cout << "* ";
}
cout << endl;
}
cout << endl << endl;
//Another pattern
for (int i{ 5 }; i >=1; --i) {
for (int j{ 1 }; j<=i; ++j) {
cout << "* ";
}
cout << endl;
}
cout << endl << endl;
//Similar to above pattern
for (int i{ 1 }; i <= 5; ++i) {
for (int j{ 1 }; j <= 5-i+1; ++j) {
cout << "* ";
}
cout << endl;
}
cout << endl << endl;
//Variations
for (int i{ 1 }; i <= 5; ++i) {
for (int j{ 1 }; j <= 5; ++j) {
if (i + j < 5) {
cout << "* ";
}
else {
cout << " ";
}
}
cout << endl;
}
cout << endl << endl;
for (int i{ 1 }; i <= 5; ++i) {
for (int j{ 1 }; j <= 5; ++j) {
if (i + j > 5) {
cout << "* ";
}
else {
cout << " ";
}
}
cout << endl;
}
cout << endl << endl;
//A triangle
for (int i{ 1 }; i <= 5; ++i) {
for (int j{ 1 }; j <= 5; ++j) {
if (i + j > 5) {
cout << "* ";
}
else {
cout << " ";
}
}
cout << endl;
}
cout << endl << endl;
//Another variation
for (int i{ 1 }; i <= 5; ++i) {
for (int j{ 1 }; j <= 5; ++j) {
if (i<j) {
cout << "* ";
}
else {
cout << " ";
}
}
cout << endl;
}
cout << endl << endl;
//Removing one space
for (int i{ 1 }; i <= 5; ++i) {
for (int j{ 1 }; j <= 5; ++j) {
if (i <=j) {
cout << "* ";
}
else {
cout << " ";
}
}
cout << endl;
}
cout << endl << endl;
//Now the Rhombus
for (int i{ 1 }; i <= 9; ++i) {
for (int j{ 1 }; j <= 9; ++j) {
if (i + j > 9) {
cout << "* ";
}
else {
cout << " ";
}
}
cout << endl;
}
//Bottom triangle
for (int i{ 1 }; i <= 9; ++i) {
for (int j{ 1 }; j <= 9; ++j) {
if (i < j) {
cout << "* ";
}
else {
cout << " ";
}
}
cout << endl;
}
cout << endl << endl;
return 0;
}
The following is the output animation from DEV-C++
Be the first to comment